If you have a website with a lot of content, it is important to ensure that your users can easily navigate through it. One way to do this is by making your website scrollable. In this article, we will go over the steps to accomplish this using the react-scroll library. With this library, you can create scrollable sections within your website and highlight the corresponding navigation items.
To get started, you will need to import the react-scroll library into your project. You can do this by adding the following code to your JavaScript file:
import Scroll from ‘react-scroll’;
const scroll = Scroll.animateScroll;
Once you have imported the library, you can make any section of your website scrollable by adding a simple scroll component. Here is an example:
This is some scrollable content.
In the example above, the scroll component is used to make the section with a corresponding id of “section1” scrollable. You can customize the smoothness and duration of the scroll by modifying the smooth and duration props.
By using the react-scroll library, you can also create a scrollable navigation bar. You can do this by combining the scroll component with the Link component. Here is an example:
import { Link } from ‘react-scroll’;
activeClass=”active”
to=”section1″
spy={true}
smooth={true}
duration={500}
>Section 1
In the example above, the Link component is used to create a link that will scroll to the section with a corresponding id of “section1”. The activeClass prop is used to highlight the currently active navigation item.
There you have it! By using the react-scroll library, you can easily make your website scrollable and provide a smooth navigation experience for your users. Just make sure to copy and paste the corresponding code examples into your project and customize them to fit your needs. Happy scrolling!
Scroll Rect
The Scroll Rect
component is a built-in Unity component that allows you to create scrollable areas within your game or application. It can be imported and exported to other projects, making it a versatile tool for creating website scrollable areas. By using the Scroll Rect
, you can make sure that the content inside the area is scrollable and accessible to users.
Here is a simple example of how to use the Scroll Rect
component:
- Add a
Scroll Rect
component to thenav
or any other section where you want to make it scrollable. - Copy and paste the
Scroll Rect
component throughout the HTML code to make sure that the corresponding areas are scrollable. - Make sure to return the
Scroll Rect
component to its original position by highlighting and copying the code snippet from theScroll Rect
component. - Import the necessary components and libraries, such as
react-scroll
or any other library you prefer, into your project to combine them with theScroll Rect
component. - If you need to create nested scrollable areas, you can use the
ms-3
class or any other class that provides the desired scrolling behavior. - Using the
Scroll Rect
component, you can also create scrollspy functionality to emphasize certain sections of your website.
By following these steps and using the Scroll Rect
component, you can make any area of your website scrollable and improve the user experience.
Example
Here is a simple example of how to make a website scrollable using the React Scroll library. In this example, we will create a scrollable area within a React project.
First, make sure you have the necessary dependencies installed. You can add the React Scroll library to your project by running the following command:
npm install react-scroll
Next, import the required components from the library:
import { Element, scroller } from 'react-scroll';
Then, create a section in your HTML code where you want the scrollable area to be:
This is the scrollable area.
In your React component, add a placeholder for the scrollable area:
function App() {
return (
- Section 1
- Section 2
- Section 3
This is the scrollable area.
);
}
export default App;
Replace the content of the scrollable area with the corresponding React Scroll components:
function App() {
return (
- Section 1
- Section 2
- Section 3
This is section 1.
This is section 2.
This is section 3.
);
}
export default App;
Finally, make sure to include the necessary CSS styles for the scrollable area:
.scrollable-area {
max-height: 200px;
overflow-y: scroll;
}
Now, your website should be scrollable. You can test it by scrolling through the sections in the scrollable area.
This is just a simple example using React Scroll, but you can also explore other features such as scroll highlighting and scrollspy to further enhance the scrolling experience on your website.
Example with nested nav
If you want to make a website scrollable throughout its entire area, you can use various methods and libraries to achieve this. One of the options is to use a scrollspy library, such as react-scroll
, which allows you to easily highlight the corresponding sections of your website as you scroll.
For this example, let’s say we are working on a project using React. First, make sure you have React and the react-scroll
library installed in your project. You can do this by running the following command:
npm install react-scroll
Once the installation is completed, you can import the necessary components:
import { Link, Element, scroller } from 'react-scroll';
Next, you need to set up the scrollable sections in your website. You can do this by adding the corresponding React components and using the Element
component from react-scroll
:
{`
Section 1
Some content goes here...
Section 2
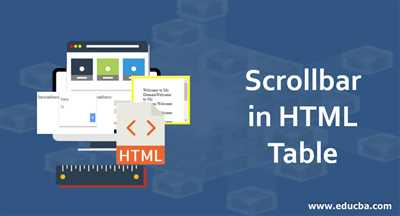
Some content goes here...
`}
Additionally, you can also add a navigation menu with links to each section using the Link
component from react-scroll
:
{``}
Make sure to set the name
attribute of each Element
component to match the to
attribute of each Link
component.
Finally, you can use the scroller
function from react-scroll
to programmatically scroll to a specific section. For example, if you want to scroll to ‘section2’, you can use the following code:
scroller.scrollTo('section2', {
smooth: true,
offset: -50,
duration: 500,
});
This is just an example of how you can make your website scrollable and emphasize the corresponding sections when scrolling. You can customize the scrolling behavior and styling to fit your needs.
Here’s a JSFiddle with the combined HTML and React code for this example:
{`
Section 1
Some content goes here...
Section 2
Some content goes here...
`}
Copy and paste this code into your HTML file or React component, and make sure to adjust the href
values and IDs to match your sections.
REACT SCROLLABLE WEBSITE
In order to create a scrollable website using React, we can utilize a library called react-scroll. This library allows us to easily implement scroll functionality to specific sections of our website. In this example, we will focus on the corresponding navigation and scroll area.
To start, make sure you have a React project set up. If you are working with a code editor, you can simply copy the code provided in this article and paste it into your project. If you prefer an online editor, you can use a platform like JSFiddle or CodeSandbox.
The first step is to install the react-scroll library. Open your terminal and navigate to your project directory. To install the library, use the following command:
npm install react-scroll
Next, you will need to import the necessary components from the library. In the file where you want to implement the scroll functionality, add the following lines:
import { Link, Element, Events, animateScroll as scroll, scrollSpy, scroller } from 'react-scroll';
This imports the required components and functions from the react-scroll library. Now, we can proceed to add the scroll functionality to our components.
In the section of your code where you want to create the scrollable area, place the following code:
This is a simple scrollable area.
You can add your content here.
Make sure to highlight the area where you want the scroll to occur.
In this example, we have used the Element component from the react-scroll library to define a scrollable area. The highlighted className can be styled according to your preference.
Now, let’s create a navigation section with links that will scroll to the corresponding sections. Add the following code:
Here, we are using the Link component to create links that will scroll to the corresponding sections when clicked. The activeClass prop allows us to apply a highlighted style to the active link. The to prop specifies the target section to scroll to.
Finally, don’t forget to export your components using:
export default YourComponent;
Throughout your project, you can now use the react-scroll library to easily implement scroll functionality. By highlighting the scrollable area and using the Link component for navigation, you can create a smooth and seamless scrolling experience for your website.
Additionally, you may also want to explore other features of the react-scroll library, such as scrollSpy, which allows you to highlight the corresponding link when scrolling through the page.
section
Sure, to make a website scrollable, you can use the “section” component. You just need to import the corresponding library, for example, using React, you can import the “react-scroll” library. This library provides a simple way to create scrollable sections in your project.
To highlight the currently active section in the navigation, you can use the “scrollspy” feature. This feature will automatically add a class to the corresponding nav element when the section is in the viewport.
Example
Here’s a simple example using the “react-scroll” library:
First, you need to import the necessary components:
import { Element, scroller } from 'react-scroll';
Then, you can use the “Element” component to create scrollable sections:
This is section 1
This is section 2
You can also use nested elements to create more complex scrollable areas. For example:
This is a nested section
To highlight the active section in the navigation, you can use the “scrollspy” feature. First, you need to set the “scrollspy” prop to true on the parent element that contains the sections:
This is section 1
This is section 2
Now, when you scroll through the sections, the corresponding nav element will be highlighted. You can also customize the highlighting by adding CSS styles to the highlighted nav element.
In this example, we used the “react-scroll” library to make a website scrollable. You can copy and paste this code into a file, and you’ll have a scrollable website with scrollspy functionality. Don’t forget to import the necessary libraries and stylesheets.
Make sure to emphasize the “name” prop on the “Element” component. This prop corresponds to the scrollspy target and should match the corresponding nav element’s “name” attribute.
Return to the top of the page
JSFiddle
In this section, we are going to highlight the usage of JSFiddle in our example. JSFiddle is a web-based platform that allows you to create, edit, and run HTML, CSS, and JavaScript code snippets in a browser. It serves as a placeholder for our code snippets and helps us visualize the scrollable website we are trying to make.
We will import some components and libraries into JSFiddle to make it work. For example, the React library will be used to create the main structure of our website. We will also need the react-scroll library for smooth scrolling between sections.
Now, let’s create a simple JSFiddle project. We’ll start by copying the corresponding HTML, CSS, and JavaScript code into the appropriate areas. Make sure to highlight the scrollable section and emphasize the scrollspy functionality implemented using React.
Here’s a simple code snippet that you can use as a starting point:
export const App = () => {
const sections = [
{
id: "section-1",
title: "Section 1",
},
{
id: "section-2",
title: "Section 2",
},
{
id: "section-3",
title: "Section 3",
},
];
return (
{sections.map((section) => (
{section.title} content goes here...
))}
);
};
In this code snippet, we have a basic React component that renders a scrollable website with three sections. Each section is represented by a
and
to display links to each section. The smooth scrolling functionality is achieved using the react-scroll
library. Make sure to go through the JSFiddle project and the corresponding code to understand how everything is combined to create a scrollable website. This will help you implement similar functionality in your own projects.